3 [](https://nodei.co/npm-dl/columnify/)
4 [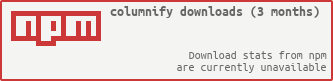](https://nodei.co/npm/columnify/)
6 [](https://travis-ci.org/timoxley/columnify)
7 [](https://npmjs.org/package/columnify)
8 [](LICENSE)
9 [](https://david-dm.org/timoxley/columnify)
10 [](https://david-dm.org/timoxley/columnify#info=devDependencies)
12 Create text-based columns suitable for console output from objects or
15 Columns are automatically resized to fit the content of the largest
16 cell. Each cell will be padded with spaces to fill the available space
17 and ensure column contents are left-aligned.
19 Designed to [handle sensible wrapping in npm search results](https://github.com/isaacs/npm/pull/2328).
21 `npm search` before & after integrating columnify:
23 
25 ## Installation & Update
28 $ npm install --save columnify@latest
34 var columnify = require('columnify')
35 var columns = columnify(data, options)
43 Objects are converted to a list of key/value pairs:
48 "minimatch@0.2.14": 3,
53 console.log(columnify(data))
64 ### Custom Column Names
69 "minimatch@0.2.14": 3,
74 console.log(columnify(data, {columns: ['MODULE', 'COUNT']}))
85 ### Columnify Arrays of Objects
87 Column headings are extracted from the keys in supplied objects.
90 var columnify = require('columnify')
92 var columns = columnify([{
109 ### Filtering & Ordering Columns
111 By default, all properties are converted into columns, whether or not
112 they exist on every object or not.
114 To explicitly specify which columns to include, and in which order,
115 supply a "columns" or "include" array ("include" is just an alias).
120 description: 'some description',
124 description: 'another description',
128 var columns = columnify(data, {
129 columns: ['name', 'version']
142 ## Global and Per Column Options
143 You can set a number of options at a global level (ie. for all columns) or on a per column basis.
145 Set options on a per column basis by using the `config` option to specify individual columns:
148 var columns = columnify(data, {
149 optionName: optionValue,
151 columnName: {optionName: optionValue},
152 columnName: {optionName: optionValue},
157 ### Maximum and Minimum Column Widths
158 As with all options, you can define the `maxWidth` and `minWidth` globally, or for specified columns. By default, wrapping will happen at word boundaries. Empty cells or those which do not fill the `minWidth` will be padded with spaces.
161 var columns = columnify([{
163 description: 'some description which happens to be far larger than the max',
167 description: 'another description larger than the max',
172 description: {maxWidth: 30}
181 NAME DESCRIPTION VERSION
182 mod1 some description which happens 0.0.1
183 to be far larger than the max
184 module-two another description larger 0.2.0
188 #### Maximum Line Width
190 You can set a hard maximum line width using the `maxLineWidth` option.
191 Beyond this value data is unceremoniously truncated with no truncation
194 This can either be a number or 'auto' to set the value to the width of
197 Setting this value to 'auto' prevent TTY-imposed line-wrapping when
198 lines exceed the screen width.
200 #### Truncating Column Cells Instead of Wrapping
202 You can disable wrapping and instead truncate content at the maximum
203 column width by using the `truncate` option. Truncation respects word boundaries. A truncation marker, `…`, will appear next to the last word in any truncated line.
206 var columns = columnify(data, {
219 NAME DESCRIPTION VERSION
220 mod1 some description… 0.0.1
221 module-two another description… 0.2.0
225 ### Align Right/Center
226 You can set the alignment of the column data by using the `align` option.
231 "commander@2.0.0": 1,
235 columnify(data, {config: {value: {align: 'right'}}})
246 `align: 'center'` works in a similar way.
249 ### Padding Character
251 Set a character to fill whitespace within columns with the `paddingChr` option.
255 "shortKey": "veryVeryVeryVeryVeryLongVal",
256 "veryVeryVeryVeryVeryLongKey": "shortVal"
259 columnify(data, { paddingChr: '.'})
264 KEY........................ VALUE......................
265 shortKey................... veryVeryVeryVeryVeryLongVal
266 veryVeryVeryVeryVeryLongKey shortVal...................
269 ### Preserve Existing Newlines
271 By default, `columnify` sanitises text by replacing any occurance of 1 or more whitespace characters with a single space.
273 `columnify` can be configured to respect existing new line characters using the `preserveNewLines` option. Note this will still collapse all other whitespace.
279 "node_modules/tap/node_modules/glob",
280 "node_modules/tape/node_modules/glob"
285 "node_modules/tap/node_modules/nopt"
288 name: "runforcover@0.0.2",
289 paths: "node_modules/tap/node_modules/runforcover"
292 console.log(columnify(data, {preserveNewLines: true}))
297 glob@3.2.9 node_modules/tap/node_modules/glob
298 node_modules/tape/node_modules/glob
299 nopt@2.2.1 node_modules/tap/node_modules/nopt
300 runforcover@0.0.2 node_modules/tap/node_modules/runforcover
303 Compare this with output without `preserveNewLines`:
306 console.log(columnify(data, {preserveNewLines: false}))
308 console.log(columnify(data))
313 glob@3.2.9 node_modules/tap/node_modules/glob node_modules/tape/node_modules/glob
314 nopt@2.2.1 node_modules/tap/node_modules/nopt
315 runforcover@0.0.2 node_modules/tap/node_modules/runforcover
318 ### Custom Truncation Marker
320 You can change the truncation marker to something other than the default
321 `…` by using the `truncateMarker` option.
324 var columns = columnify(data, {
338 NAME DESCRIPTION VERSION
339 mod1 some description> 0.0.1
340 module-two another description> 0.2.0
343 ### Custom Column Splitter
345 If your columns need some bling, you can split columns with custom
346 characters by using the `columnSplitter` option.
349 var columns = columnify(data, {
350 columnSplitter: ' | '
357 NAME | DESCRIPTION | VERSION
358 mod1 | some description which happens to be far larger than the max | 0.0.1
359 module-two | another description larger than the max | 0.2.0
362 ### Control Header Display
364 Control whether column headers are displayed by using the `showHeaders` option.
367 var columns = columnify(data, {
372 This also works well for hiding a single column header, like an `id` column:
374 var columns = columnify(data, {
376 id: { showHeaders: false }
381 ### Transforming Column Data and Headers
382 If you need to modify the presentation of column content or heading content there are two useful options for doing that: `dataTransform` and `headerTransform`. Both of these take a function and need to return a valid string.
385 var columns = columnify([{
387 description: 'SOME DESCRIPTION TEXT.'
390 description: 'SOME SLIGHTLY LONGER DESCRIPTION TEXT.'
392 dataTransform: function(data) {
393 return data.toLowerCase()
397 headingTransform: function(heading) {
398 heading = "module " + heading
399 return "*" + heading.toUpperCase() + "*"
407 *MODULE NAME* DESCRIPTION
408 mod1 some description text.
409 module-two some slightly longer description text.
413 ## Multibyte Character Support
415 `columnify` uses [mycoboco/wcwidth.js](https://github.com/mycoboco/wcwidth.js) to calculate length of multibyte characters:
420 description: 'some description',
423 name: '这是一个很长的名字的模块',
424 description: '这真的是一个描述的内容这个描述很长',
428 console.log(columnify(data))
431 #### Without multibyte handling:
433 i.e. before columnify added this feature
436 NAME DESCRIPTION VERSION
437 module-one some description 0.0.1
438 这是一个很长的名字的模块 这真的是一个描述的内容这个描述很长 0.3.3
441 #### With multibyte handling:
444 NAME DESCRIPTION VERSION
445 module-one some description 0.0.1
446 这是一个很长的名字的模块 这真的是一个描述的内容这个描述很长 0.3.3
453 repo age : 1 year, 2 months
465 1 Isaac Z. Schlueter 0.8%